漂亮骚妇黑丝勾引外卖哥先插b,再爆菊花,内射。_x264_aac
发布于
  7496
热门国产:
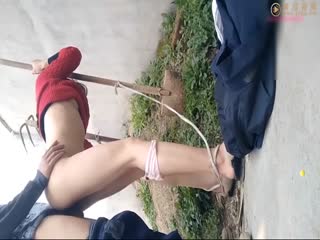
热播
白天来一发晴天白日炮
8346 556
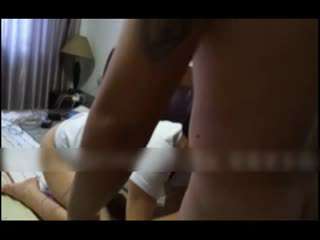
热播
身材漂亮模特人廋胸大浴室自慰很是诱惑高清
8704 463
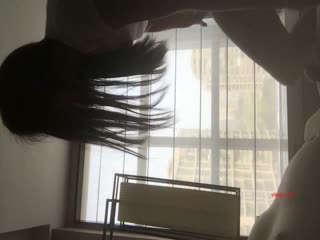
热播
[高质量国产精品]泄密流出精彩视频情侣间的有料自拍7V合1
6687 723
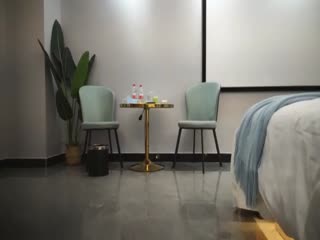
热播
[91第一深情也叫日久深情],高端外围 经骨好软的妹子 仙丹加持 凹腿狂操 两回合
8057 875
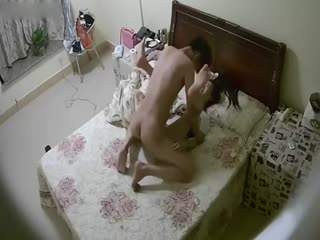
热播
偷拍小两口过夫妻生活老公卖力耕耘老婆忙着玩手机
5948 664
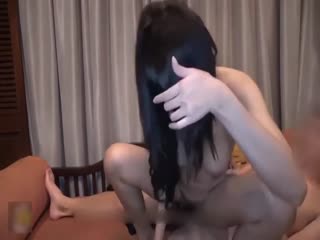
热播
白嫩G奶爆乳极品女神,各种姿势满足小骚货快速抽插
8176 686
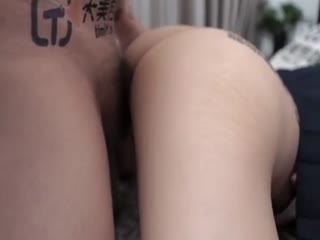
热播
巨乳少妇成了我的室友.x264.aac
5767 615

热播
手机直播美女主播和帅哥炮友啪啪大秀69互舔多种姿势爆操
7754 737
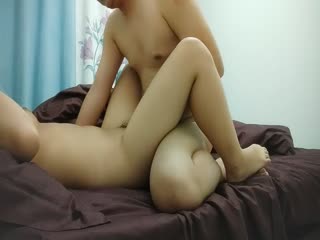
热播
探花小队长_20210901
9087 362
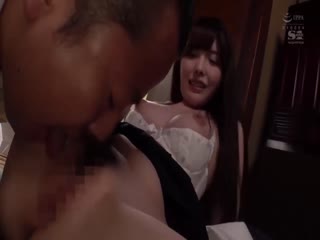
热播
桥本制服腿玩年1
4725 942
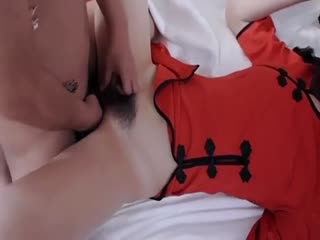
热播
星空无限传媒元旦庆典_ 女优狂欢啪.x264.aa
9463 749
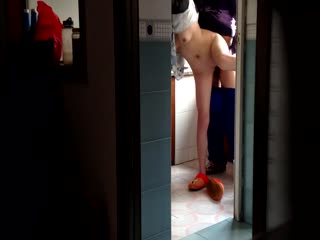
热播
国产主播新[43]
6188 340
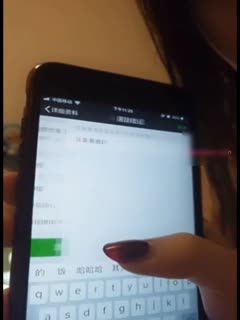
热播
花椒性感女神主播萌鹿鹿為了打賞大秀福利
4294 692
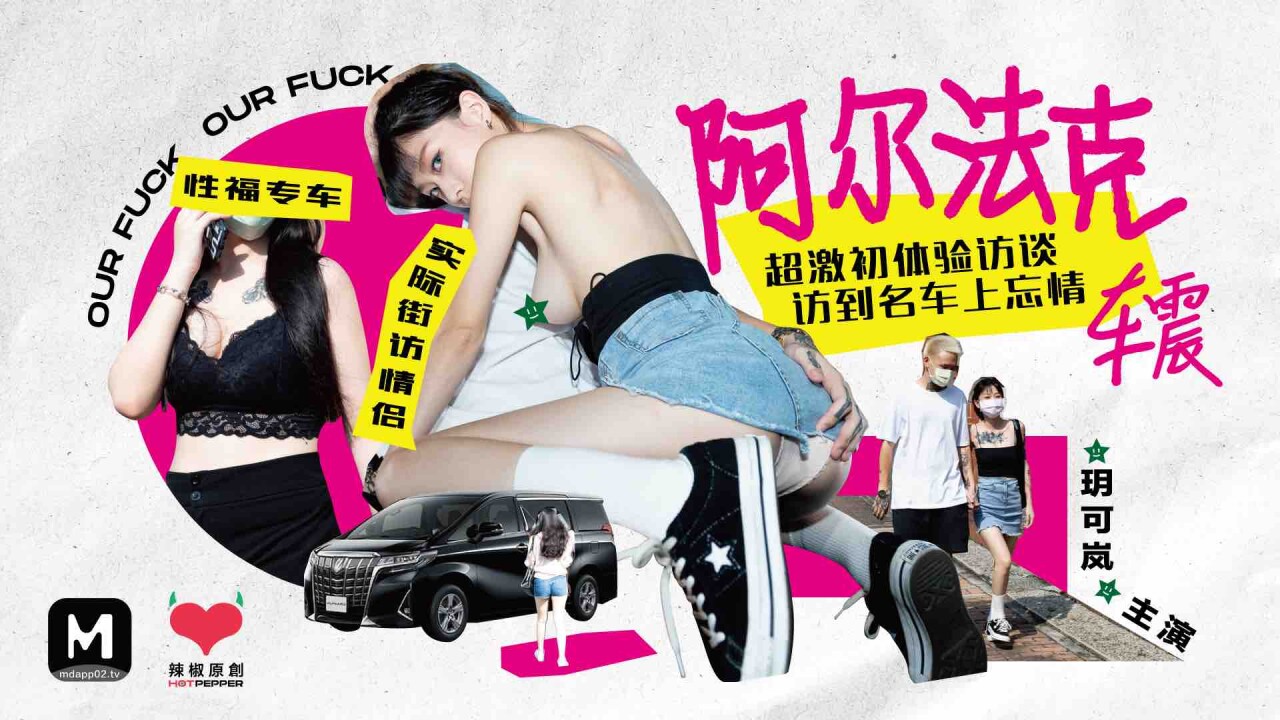
热播
國產麻豆AV 番外 辣椒原創 HPP 阿爾法克 超激初體驗訪談訪到名車上忘情車震 玥可嵐
8512 165
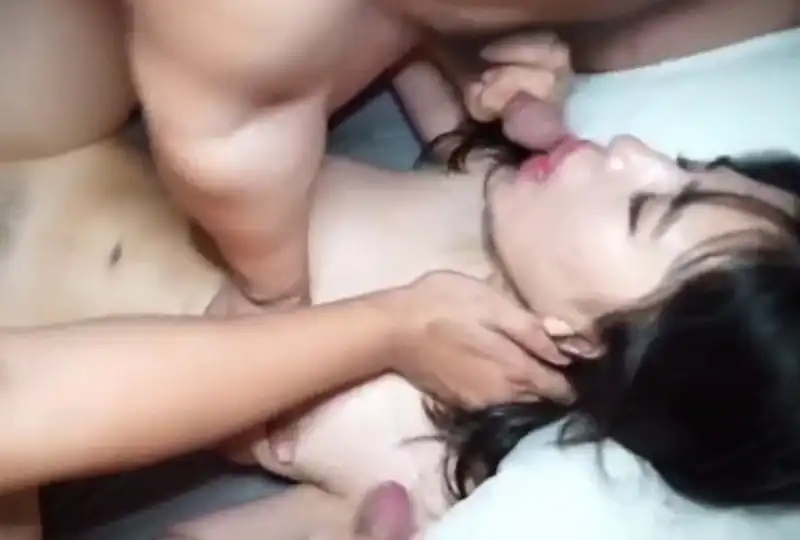
热播
无法满足性欲的痴女要求多位男人玩弄她身体
7636 955
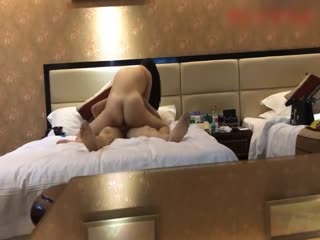
热播
宾馆约炮约到巨乳女大生
1798 536
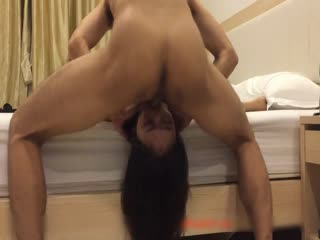
热播
真实自拍17私藏作品-杭州白嫩性感漂亮美女和闺蜜男友酒
2662 530
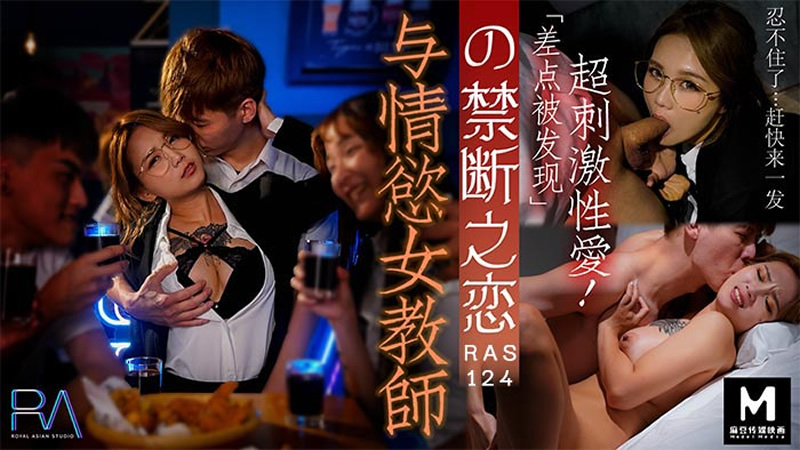
热播
与情欲女教师的禁断之恋[皇家华人 HJ-089]
5230 855
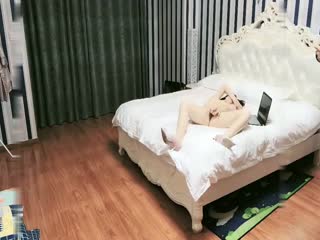
热播
22岁艺校女生被两个社会哥冒充摄影师套路到酒店私拍潜规
9929 923
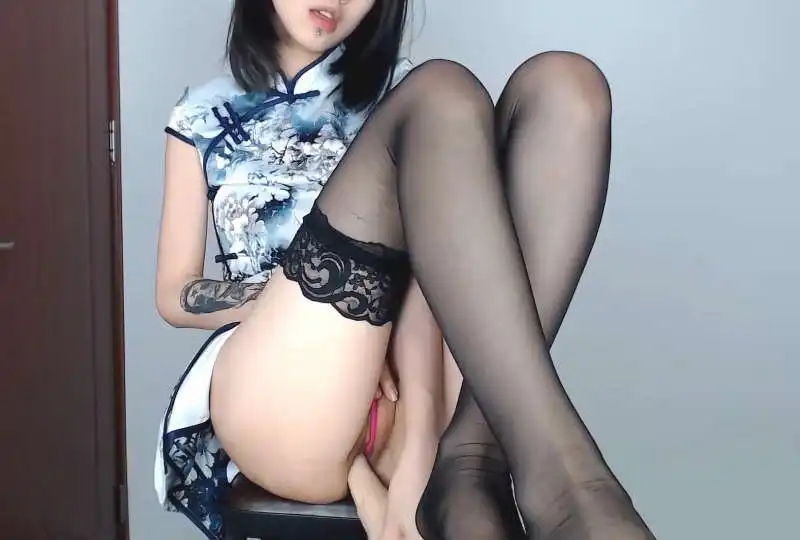
热播
没看过这么特别的乳头 旗袍美妹子道具自慰
1299 598
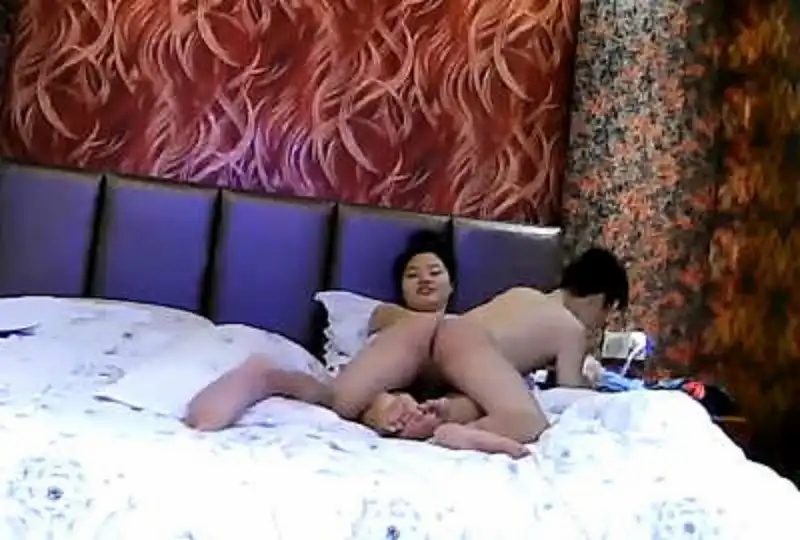
热播
大学生情侣很激情 忍不住在沙发上直接操
1427 126
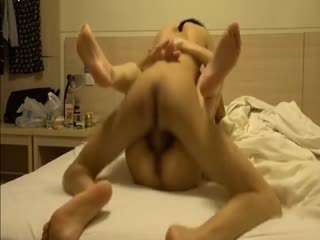
热播
Chinese炮友约炮中1
9065 614
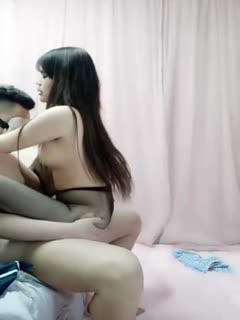
热播
白嫩美乳红唇妹子两男一女3P淫乱,边舔屌口交边被后入,上位骑坐抽插两男轮换着操_x264_aac
9892 750
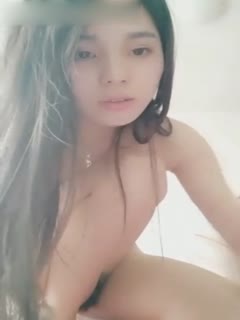
热播
夫妻口交秀床上快速吸吮高潮射精掰开逼逼很是诱惑喜欢不要
1916 920
热门推荐
推荐